Digital Signature is widely used to protect the PDF files and it is so important that we always try our best to improve our Spire.PDF better and better. Besides the previous method of creating digital signature in PDF, Spire.PDF now supports to create visible digital signature in PDF with C# code.
Make sure Spire.PDF for .NET (version 2.9 or above) has been installed correctly. Add Spire.PDF.dll as reference in the downloaded Bin folder through the below path: "...\Spire.PDF\Bin\NET4.0\ Spire.PDF.dll".
02 | using Spire.Pdf.Graphics; |
03 | using Spire.Pdf.Security; |
07 | namespace DigitalSignature |
11 | static void Main( string [] args) |
14 | PdfDocument doc = new PdfDocument(); |
15 | doc.LoadFromFile( @"C:\Users\Administrator\Desktop\test.pdf" ); |
18 | PdfCertificate cert = new PdfCertificate( @"C:\Users\Administrator\Desktop\gary.pfx" , "e-iceblue" ); |
21 | PdfSignature signature = new PdfSignature(doc, doc.Pages[0], cert, "Signature1" ); |
24 | signature.Bounds = new RectangleF( new PointF(200, 200), new SizeF(200, 90)); |
27 | signature.SignImageSource = PdfImage.FromFile( @"C:\Users\Administrator\Desktop\E-iceblueLogo.png" ); |
30 | signature.GraphicsMode = GraphicMode.SignImageAndSignDetail; |
31 | signature.NameLabel = "Signer:" ; |
32 | signature.Name = "Gary" ; |
33 | signature.ContactInfoLabel = "ContactInfo:" ; |
34 | signature.ContactInfo = signature.Certificate.GetNameInfo(System.Security.Cryptography.X509Certificates.X509NameType.SimpleName, true ); |
35 | signature.DistinguishedNameLabel = "DN: " ; |
36 | signature.DistinguishedName = signature.Certificate.IssuerName.Name; |
37 | signature.LocationInfoLabel = "Location:" ; |
38 | signature.LocationInfo = "Chengdu" ; |
39 | signature.ReasonLabel = "Reason: " ; |
40 | signature.Reason = "Le document est certifie" ; |
41 | signature.DateLabel = "Date:" ; |
42 | signature.Date = DateTime.Now; |
43 | signature.DocumentPermissions = PdfCertificationFlags.AllowFormFill | PdfCertificationFlags.ForbidChanges; |
44 | signature.Certificated = true ; |
47 | signature.SignDetailsFont = new PdfFont(PdfFontFamily.TimesRoman, 10f); |
48 | signature.SignNameFont = new PdfFont(PdfFontFamily.Courier, 15); |
51 | signature.SignImageLayout = SignImageLayout.None; |
54 | doc.SaveToFile( "signature.pdf" ); |
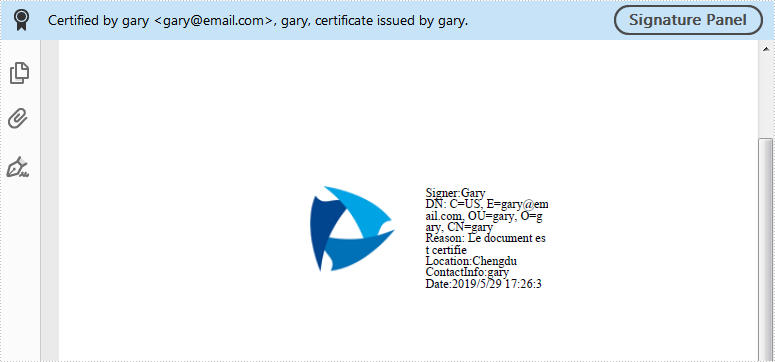
No comments:
Post a Comment