Introduction:
Now in this article I am going to explain with example how to validate CheckBoxList control i.e. to ensure at least one item is selected in the CheckBoxList. I am using CustomValidatorcontrol and JavaScript to validate.
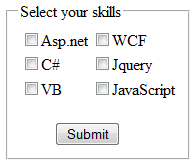
Implementation: Let's understand by an practical example.
Now in this article I am going to explain with example how to validate CheckBoxList control i.e. to ensure at least one item is selected in the CheckBoxList. I am using CustomValidatorcontrol and JavaScript to validate.
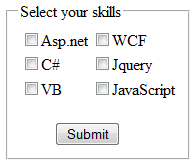
Implementation: Let's understand by an practical example.
- In the <HEAD> tag of the design page(.aspx) create the JavaScript function to validate the CheckBoxList.
function validateCheckBoxList(source, args) {
var chkListModules = document.getElementById('<%= cblCourses.ClientID %>');
var chkListinputs = chkListModules.getElementsByTagName("input");
for (var i = 0; i < chkListinputs.length; i++) {
if (chkListinputs[i].checked) {
args.IsValid = true;
return;
}
}
args.IsValid = false;
}
</script>
- In the <Body> tag place a CheckBoxList, a Label control, a Button control and a CustomValidator control. In the CheckBoxList add some items as shown below:
<fieldset style="width:160px">
<legend>Select your skills</legend>
<asp:CheckBoxList ID="cblCourses" runat="server" RepeatColumns="2">
<asp:ListItem>Asp.net</asp:ListItem>
<asp:ListItem>C#</asp:ListItem>
<asp:ListItem>VB</asp:ListItem>
<asp:ListItem>WCF</asp:ListItem>
<asp:ListItem>Jquery</asp:ListItem>
<asp:ListItem>JavaScript</asp:ListItem>
</asp:CheckBoxList>
<asp:Label ID="lblStatus" runat="server" Text=""></asp:Label>
<asp:CustomValidator ID="CustomValidator1" runat="server" ErrorMessage="Please select at least one skills."ClientValidationFunction = "validateCheckBoxList" Display="static" ForeColor="Red"></asp:CustomValidator>
<asp:Button ID="btnSubmit" runat="server" Text="Submit"
onclick="btnSubmit_Click" />
</fieldset>
C#.Net Code to validate CheckBoxList using JavaScript
- In the code behind file(.aspx.cs) write the code as:
protected void btnSubmit_Click(object sender, EventArgs e)
{
lblStatus.Text="Validation successful";
}
VB.Net Code to validate CheckBoxList using JavaScript
- In the code behind file(.aspx.vb) write the code as:
lblStatus.Text = "Validation successful"
End Sub
No comments:
Post a Comment